Auto Empire: Engineering a Dealership Dynasty with C++ Classes
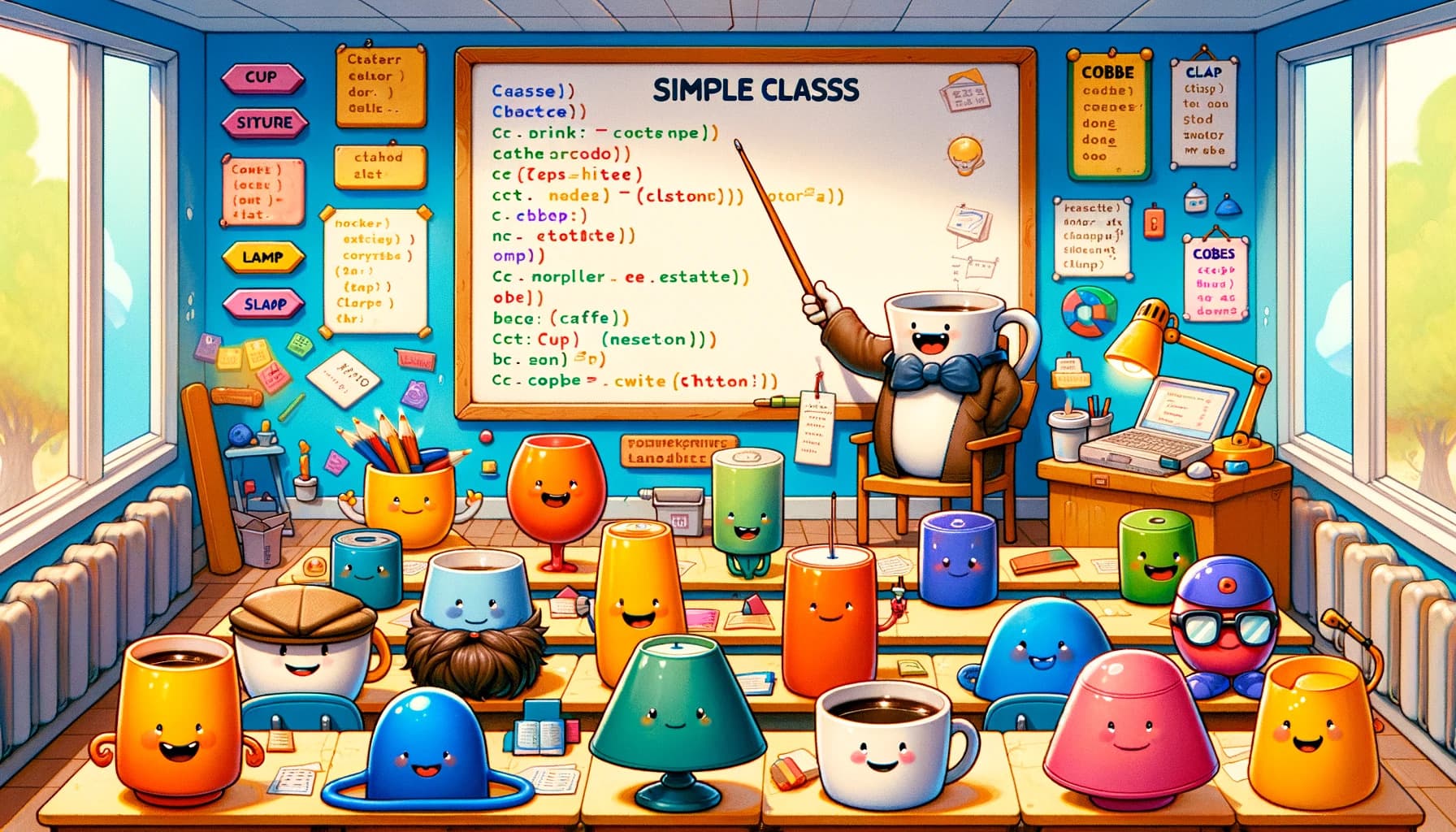
Welcome to the grand showroom of Lab 1: Classes, where the rubber of object-oriented programming meets the road of C++. Here, in the bustling metropolis of code, lies a dealership not of cars, but of knowledge, waiting for aspiring engineers to take the wheel. Whether you're a rookie racer in the world of C++ or a seasoned driver on the OOP track, this project is your ticket to mastering the fundamentals of classes, objects, and more.
For source code please visit the Github repository here: Data Visualization
Overview
Lab 1: Classes is a C++ project designed to illustrate the fundamental concepts of object-oriented programming, including class definition, object instantiation, and basic operations on objects. The project simulates a simple dealership management system, manipulating vehicles and showrooms.
Repository Structure
Lab 1.pdf
: Documentation providing an overview and specifications for the lab project.Dealership.cpp
&Dealership.h
: Defines the Dealership class, managing a collection of Showroom objects.Main.cpp
&Main.h
: Contains the main driver code that demonstrates the usage of Vehicle, Showroom, and Dealership classes.Showroom.cpp
&Showroom.h
: Implements the Showroom class, managing a collection of Vehicle objects.Vehicle.cpp
&Vehicle.h
: Defines the Vehicle class, representing individual vehicles with attributes like make, model, year, price, and mileage.
Key Functionalities
Vehicle Class
The Vehicle class encapsulates data about a vehicle, including its make, model, year, price, and mileage. It provides methods to display vehicle information and access vehicle attributes.
- Constructor Overloading: Supports creating a Vehicle object with default values or specified attributes.
- Display Information: A method to neatly print the vehicle's details.
Showroom Class
The Showroom class represents a showroom that can hold multiple vehicles. It allows adding vehicles to the showroom and displays all vehicles within it.
- Capacity Management: Limits the number of vehicles in a showroom based on its capacity.
- Add Vehicle: Adds a vehicle to the showroom if it has not reached its capacity.
- Show Inventory: Lists all vehicles in the showroom, including their details.
Dealership Class
The Dealership class manages multiple showrooms. It demonstrates the aggregation relationship in OOP, where a dealership aggregates several showrooms.
- Add Showroom: Incorporates a new showroom into the dealership.
- Show Inventory: Displays the inventory of all showrooms under the dealership, including each vehicle's details.
- Average Price Calculation: Computes the average price of all vehicles across all showrooms in the dealership.
Example Usage
The main function (Main.cpp
) demonstrates the usage of these classes through a series of tests:
- Creating Vehicles: Instantiates Vehicle objects with different attributes.
- Managing a Showroom: Adds vehicles to a showroom and displays the showroom inventory.
- Dealership Operations: Creates a dealership, adds showrooms to it, and displays the entire dealership's inventory.
Conclusion
As the garage door closes on Lab 1: Classes, we step out, not just with a dealership forged in the fires of C++, but with a toolbox brimming with the essentials of object-oriented programming. This journey through the world of classes, objects, and aggregation is more than a lesson—it's a launchpad into the universe of software development, where every line of code is a road leading to new adventures. So buckle up, programmers, for the road ahead is paved with opportunities to innovate, create, and explore the endless highways of coding.