Charting the Data Seas: Navigating STL Maps in C++
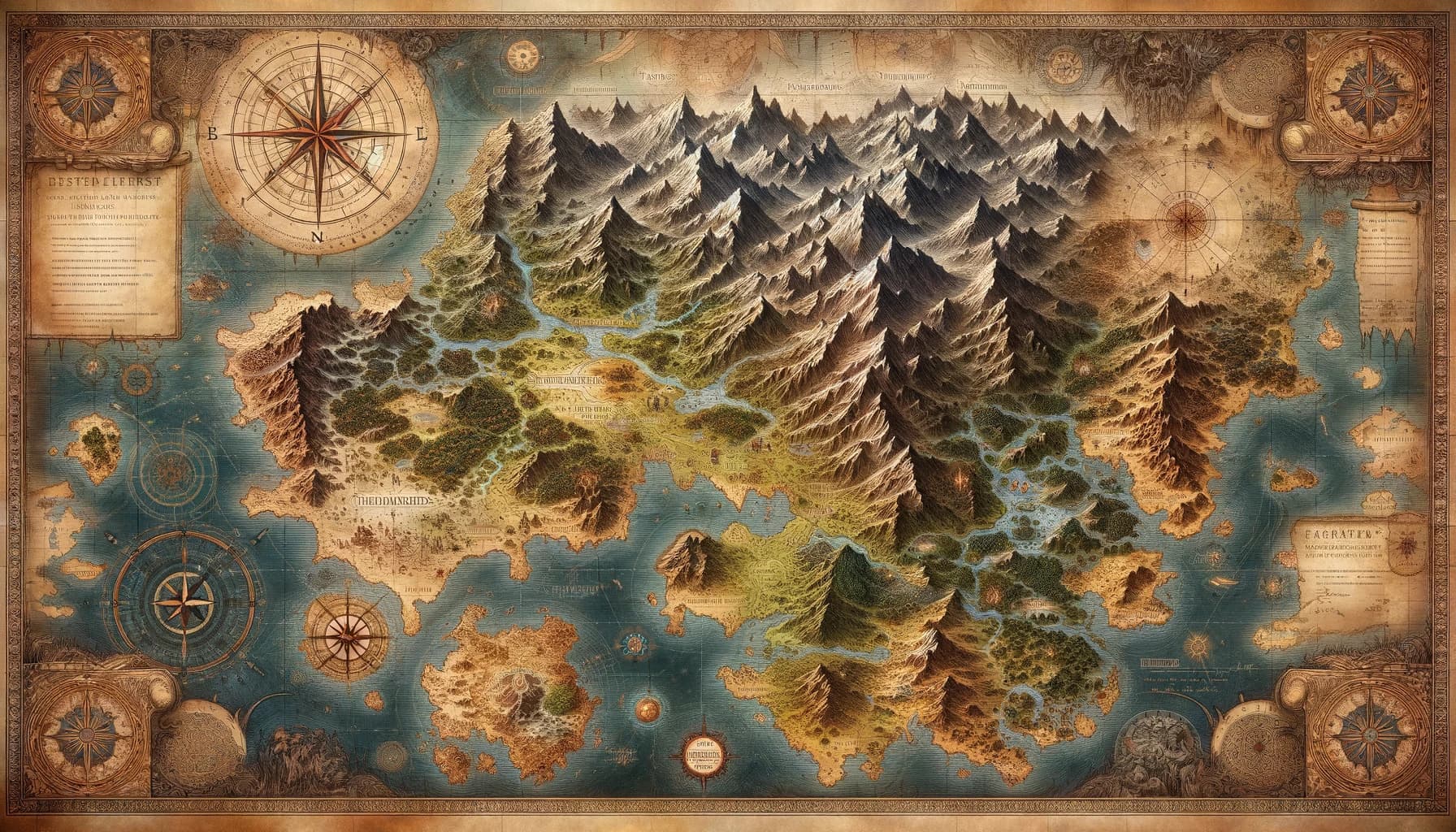
In the uncharted territories of C++ programming, there lies a map that promises to guide intrepid explorers through the dense forests of data: Lab 7: Maps. This voyage is not for the faint-hearted, as it delves into the mysteries of the Standard Template Library's (STL) map data structure, armed with the compass of real-world demographic data and the sextant of random number generation.
For source code please visit the Github repository here: Maps
Overview
Lab 7: Maps is a C++ project designed to illustrate the usage of the Standard Template Library's (STL) map data structure in conjunction with real-world data. The project includes a main application (main.cpp
) that performs operations such as random number generation and manipulation of state demographic data stored in a CSV file (states.csv
).
Repository Structure
main.cpp
: The core application file that includes the implementation of random number generation and the functionality to load and query state demographic data using an STL map.main.h
: The header file formain.cpp
, potentially intended to declare any functions or classes used withinmain.cpp
.states.csv
: A CSV file containing demographic data for various states, including information on per capita income, population, median household income, and the number of households.Documentation/[1] Ex1 - Scientific Calculator.pdf
: Provides comprehensive documentation on the project, including specifications and user instructions.
Key Functionalities
Random Number Generation
The application demonstrates a simple use case of generating random numbers within a specified range. This functionality is designed to showcase how random number generation can be implemented in C++.
State Demographic Data Management
The core feature of this project is the manipulation of state demographic data using an STL map. Key functionalities include:
- Data Loading: The application loads state data from the
states.csv
file into an STL map, where each state's name is the key, and its demographic data is stored in a States class instance. - Querying Data: Users can query demographic information for all states or a specific state, displaying data such as population, per capita income, median household income, and the number of households.
States Class
Defined within main.cpp
, the States class encapsulates the demographic data for a state. It includes methods for accessing this data, such as:
- Getters: Methods like
GetState()
,GetPopulation()
,GetCapita()
,GetHouseholdIncome()
, andGetNumHouse()
provide access to the state's demographic attributes.
User Interaction
The application offers a simple command-line interface that allows users to select between generating random numbers and querying state demographic data. It further allows users to choose between displaying data for all states or searching for a specific state.
Usage
- Compile the application using a C++ compiler that supports C++11 or later.
- Run the compiled executable. Follow the on-screen prompts to choose between random number generation and demographic data queries.
- For demographic data, you can either display information for all states or enter a state name to get specific data.
Conclusion
As our voyage with Lab 7: Maps comes to an anchor, we find ourselves enriched with the lore of STL maps, the wisdom of data manipulation, and the thrill of discovery. This project stands as a beacon for those who seek to master the art of data handling in C++, a testament to the power of maps in charting the vast oceans of information. So, with the compass of STL maps in hand, to what new adventures will you set your course? The world of data awaits.