Shape Shifters Unleashed: Mastering Inheritance in C++
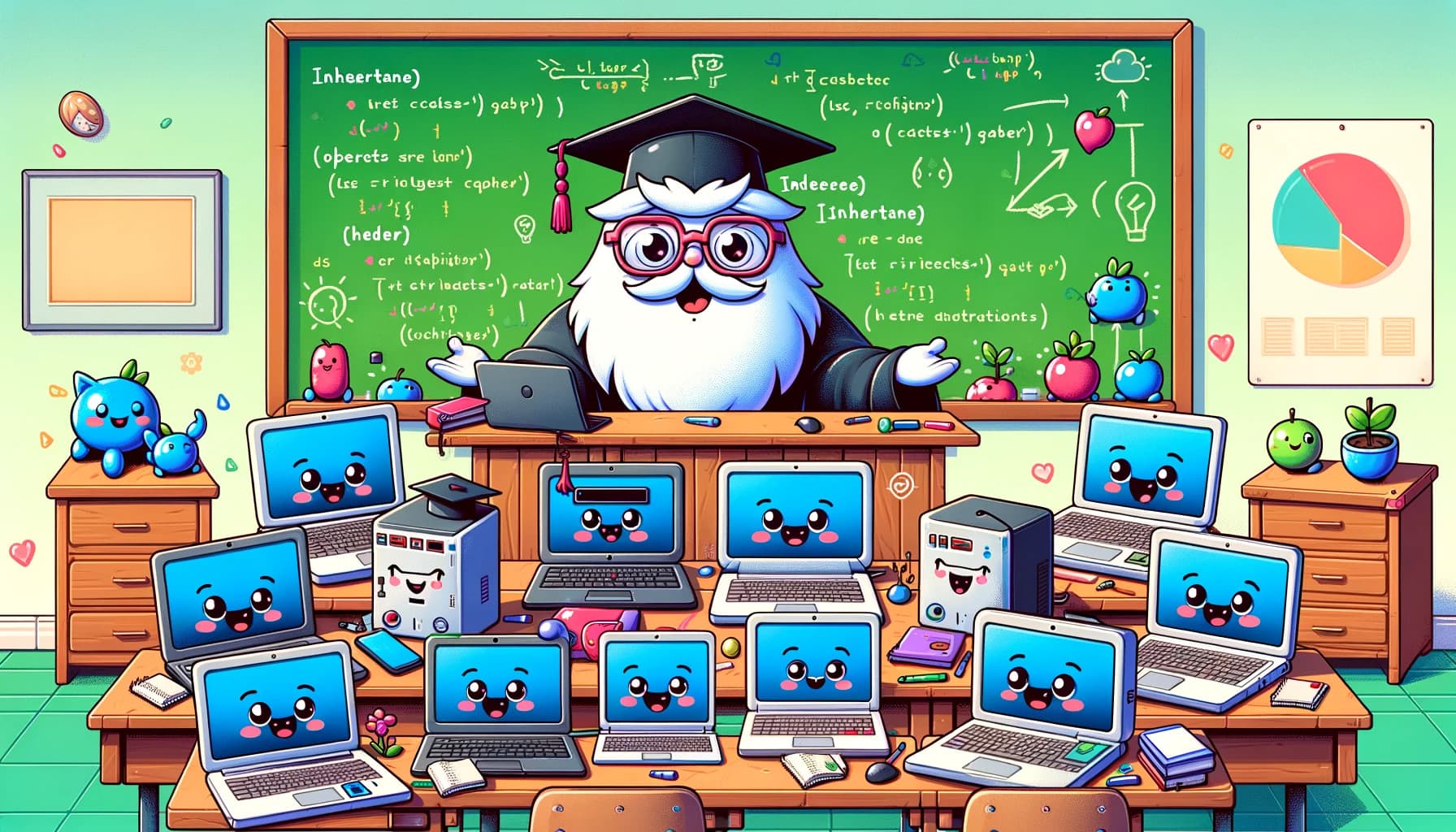
In the alchemist's lab of C++ programming, there exists a tome of knowledge so potent that it can breathe life into the abstract, morphing mere concepts into tangible entities. This is the tale of Lab 6: Inheritance, a saga where two-dimensional and three-dimensional shapes transcend their mathematical confines, thanks to the sorcery of inheritance, the versatility of polymorphism, and the cunning of operator overloading.
For source code please visit the Github repository here: Inheritance
Overview
Lab 6: Inheritance is a C++ project that demonstrates the use of inheritance, polymorphism, and operator overloading through the implementation of various geometric shapes. The project consists of a series of classes that represent two-dimensional (2D) and three-dimensional (3D) shapes, showcasing the power of object-oriented programming (OOP) principles in C++.
Repository Structure
Shapes.cpp
andShapes.h
: These files contain the definitions and implementations of the shape classes, includingShape
,Shape2D
,Shape3D
, and specific shapes likeSquare
,Triangle
,Circle
,Sphere
,Cylinder
, andTriangularPyramid
. They demonstrate inheritance, withShape2D
andShape3D
inheriting fromShape
, and the specific shapes inheriting from these two classes as appropriate.main.cpp
andmain.h
: Themain.cpp
file contains the main driver code for the application, showcasing various tests to demonstrate the functionality of the shape classes, including construction, area and volume calculation, display, and scaling.Lab 6.vcxproj
: The Visual Studio project file used to compile and run the C++ code.Lab 6.pdf
: Documentation that provides additional context and requirements for the lab project.
Key Functionalities
Shape Hierarchy
- Base Class Shape: Abstract base class that defines common functionalities for all shapes.
- Derived Classes Shape2D and Shape3D: Serve as base classes for two-dimensional and three-dimensional shapes, respectively, introducing methods for area (
Area()
) and volume (Volume()
) calculations. - Concrete Shape Classes: Implement specific shapes and override the
Area()
,Volume()
, andDisplay()
methods as appropriate. They also include aScale()
method to adjust the size of the shape.
Polymorphism and Operator Overloading
- Polymorphism: Demonstrated through the use of virtual functions and inheritance, allowing shapes to be manipulated and displayed polymorphically.
- Operator Overloading: The classes include overloaded comparison operators (
>
,<
,==
) to compare shapes based on their area or volume.
Test Functions
- TestOne through TestFive: A series of test functions defined in
main.cpp
that demonstrate various functionalities, such as testing constructors, scaling shapes, and comparing shapes based on area or volume.
Random Shape Generation
- RandomShapes, Random2DShapes, and Random3DShapes: Functions that generate random shapes with random dimensions to test the scalability and comparison operators.
Usage
To run the project:
- Compile the project using Visual Studio or a compatible C++ compiler that supports the project configuration.
- Execute the compiled program. Input prompts in
main.cpp
guide the user through selecting specific tests to run.
Conclusion
As we close the chapter on Lab 6: Inheritance, what remains is a realm transformed, where shapes no longer conform to the boundaries of imagination but leap forth, vibrant and alive, from the depths of C++. This journey through the hierarchy of shapes not only illuminates the path of inheritance and polymorphism but also lays bare the infinite possibilities that await when code transcends its mortal coil to shape the very fabric of reality. So, noble coder, with the power of C++ inheritance at your command, what worlds will you build? What shapes will you shift? The canvas of creation is yours to command.