The Adventure of Building a Linked List in C++
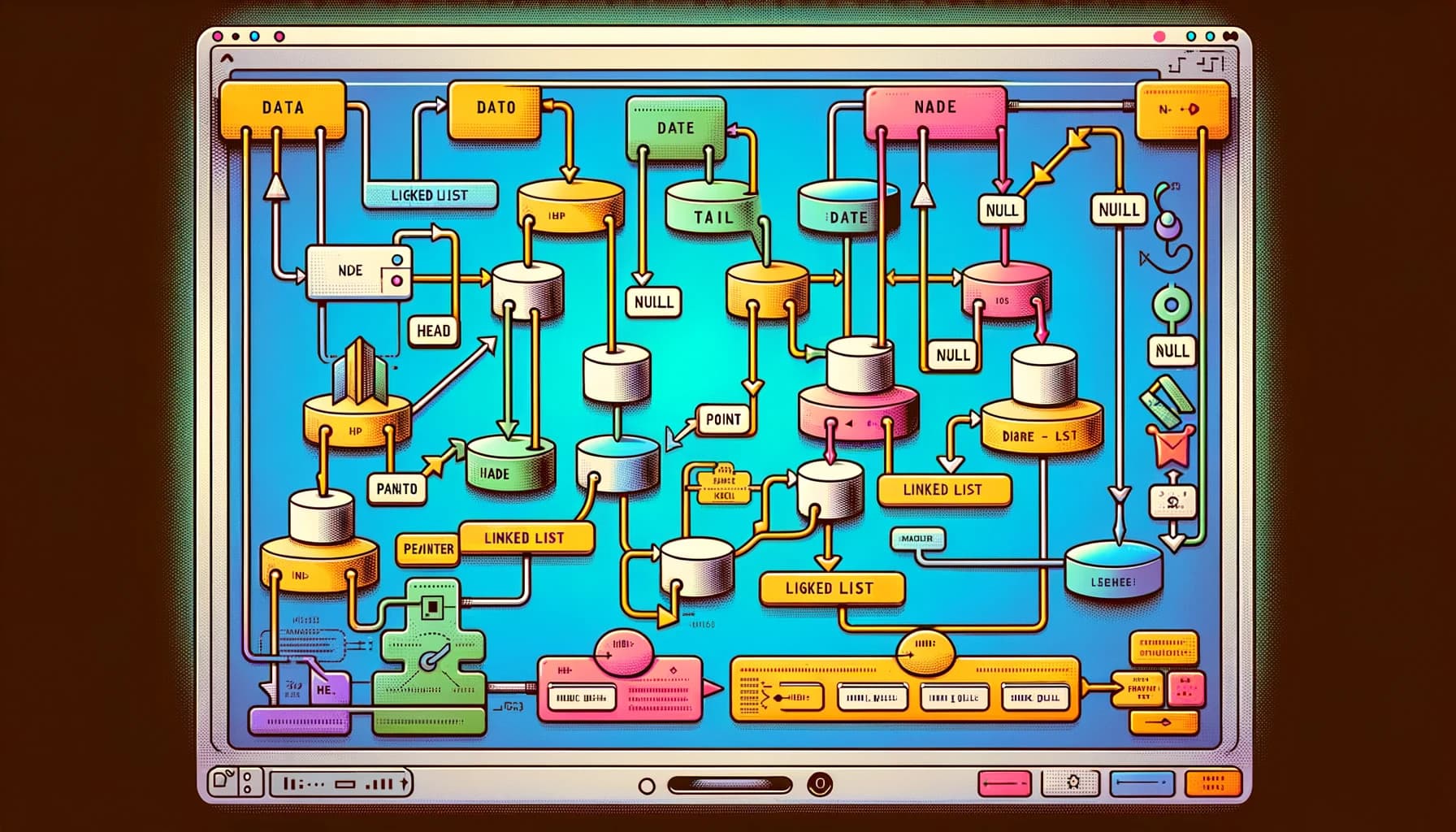
In a digital world where data is the new gold, efficient management and manipulation of information are paramount. Enter the realm of linked lists, a fundamental structure in the heart of computer science. Our journey through "Linked Lists" unveils the elegance of nodes linked in a dance of pointers, each step meticulously coded in C++. Armed with a compiler that whispers the language of C++17 and the SFML library as our canvas, we embark on an adventure to reimagine data storage and retrieval.
For source code please visit the Github repository here: Linked Lists
Exploring the Intricacies of Linked Lists with C++ and SFML
The Linked Lists repository is a treasure trove for anyone delving into the world of data structures using C++. This project not only demonstrates the practical implementation of a linked list but also leverages the Simple and Fast Multimedia Library (SFML) for an enriched learning experience. Below is a detailed exploration of the repository's contents, focusing on source code, project configuration, and documentation.
Overview
The project is structured with source code files defining the linked list and its operations, project configuration files for Visual Studio, debug and build logs, documentation, and compiled object files.
Source Code and Headers
-
LinkedList.cpp
&LinkedList.h
: These files form the backbone of the linked list implementation. They define a templatedLinkedList
class encapsulating all functionality associated with the data structure, including node creation, list manipulation, and destruction processes. TheNode
struct defined within represents the elements of the list, comprising data of a generic typeT
and pointers to the next and previous nodes. -
main.cpp
&main.h
:main.cpp
acts as the driver for the project, containing a suite of test functions that instantiate theLinkedList
class to demonstrate various operations such as element addition, removal, and searching within the list.main.h
provides the declarations for these test functions. -
leaker.cpp
&leaker.h
: Intended for memory management and debugging purposes, these files likely include tools or mechanisms to detect memory leaks and ensure efficient memory usage throughout the linked list operations.
Project Configuration and Build Files
The project includes Visual Studio configuration files (*.vcxproj
, *.vcxproj.filters
, and *.vcxproj.user
) that contain settings for compilation and project dependencies, facilitating the project's setup and execution in a Visual Studio environment.
Debug and Build Logs
Generated during the build process, debug directories and files (such as .tlog
extensions) contain logs that are essential for diagnosing and resolving build issues.
Documentation
LinkedList.pdf
: Presumed to contain theoretical explanations and detailed descriptions of the linked list implementation, this document serves as a comprehensive guide for users and contributors to understand the intricacies of the project.
Executable and Object Files
Compiled object files (.obj
) and potentially encrypted or encoded versions of these files (.enc
) represent the executable components of the project, ready for deployment or further testing.
Core Functionalities
The LinkedList
class implements a wide array of functionalities, essential for the manipulation and management of linked lists. These include:
- Element Insertion: Methods for adding elements at the beginning (
AddHead
), end (AddTail
), or at a specified position within the list. - Element Deletion: Capabilities to remove elements from specific positions, or based on their value, with additional support for clearing the entire list.
- List Traversal: Functions for iterating over the list elements, either sequentially or recursively, and in both forward and reverse directions.
- Element Searching: Implementation of search operations to locate elements by their value within the list.
Conclusion: A Tale of Nodes and Connections
As our odyssey through the linked list landscape concludes, we stand in awe of the structures we've built, node by node, pointer by pointer. "Linked Lists" is more than a foray into data structures; it's a saga of connections made and broken, of information stored and retrieved, all within the vast expanse of memory managed by our code.
In this digital age, where data's value is immeasurable, understanding and implementing structures like linked lists is not just academic—it's a rite of passage for any programmer seeking to master the art of coding. So here's to the linked lists we've built and the adventures yet to come in the ever-expanding universe of computer science.