Painting with Code: A C++ Odyssey into Hexadecimal Colors
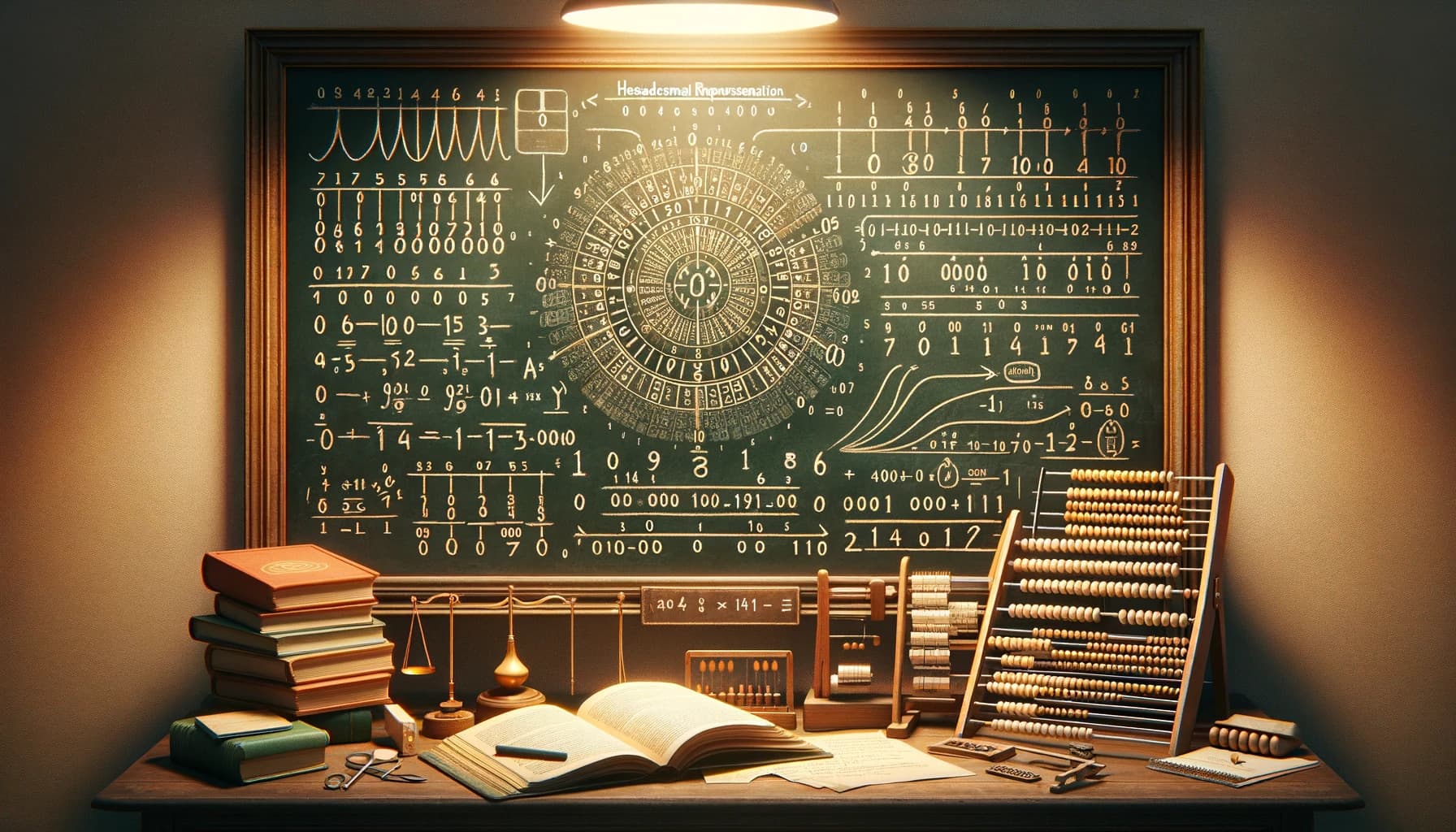
Welcome to a technicolor journey through the "Lab-8-Hexadecimal-Representation" project, where the hues of innovation blend with the palette of C++ programming. Imagine a world where every color you see can be translated into the language of code, creating a bridge between the digital and the visual. This project is your canvas, and C++ is your brush, ready to paint the pixels with the precision of hexadecimal values.
For source code please visit the Github repository here: Hexadecimal Representation
Components Overview
Source Files
-
Color.cpp
&Color.h
: Define theColor
class, responsible for color object creation and management. This class includes methods for setting color values, converting between RGB and hexadecimal formats, and retrieving color attributes. -
main.cpp
: Implements the application's main logic. It reads color definitions from text files, converts them toColor
objects, and provides an interface for user interaction, such as displaying all colors or searching for specific colors by name.
Color Class
Constructors and Properties
- The
Color
class constructor initializes color properties including RGB values, the color name, and its hexadecimal representation. - Private member variables store the color's RGB values, name, and hexadecimal string.
Public Methods
SetValue(int value)
: Converts an integer value to its RGB components and updates the object's hexadecimal string representation accordingly.SetName(const char* name)
: Sets the color's name.GetR()
,GetG()
,GetB()
: Return the red, green, and blue components of the color, respectively.GetHexValue()
: Returns the color's hexadecimal representation.GetName()
: Returns the color's name.
Main Application Logic
- The
main
function presents a menu for loading color definitions from one or more text files (colors1.txt
,colors2.txt
, etc.), displaying all loaded colors, or searching for a specific color by name. ReadFile(const char* filename, map<string, Color>& colors)
: Reads color definitions from a specified file, creatingColor
objects for each definition and storing them in a map keyed by color name.PrintColors(const map<string, Color>& colors)
: Iterates through the map of colors and prints each one, showing its name, hexadecimal value, and RGB components.SearchForColor(map<string, Color>& colors)
: Prompts the user to enter a color name, searches the map for the color, and prints its details if found.
Utility and Sample Data Files
colors1.txt
&colors2.txt
: Contain sample color definitions used by the application. Each line in these files specifies a color name followed by its integer value, which is then converted into RGB and hexadecimal formats by the application.
Usage
To use the application, compile the source files into an executable, run the program, and follow the on-screen prompts. Users can load color definitions from provided text files, view all loaded colors, and search for specific colors by name.
Conclusion
The "Lab-8-Hexadecimal-Representation" project is more than a C++ application; it's a journey into the heart of color representation, blending hexadecimal values and RGB formats into a spectrum of possibilities. Through this exploration, we not only learn the mechanics of color conversion but also appreciate the role of programming in painting our digital world with endless hues. So here's to the colors yet to be coded, and the projects yet to be painted.