Interstellar Chronicles: Navigating the Binary Universe with C++
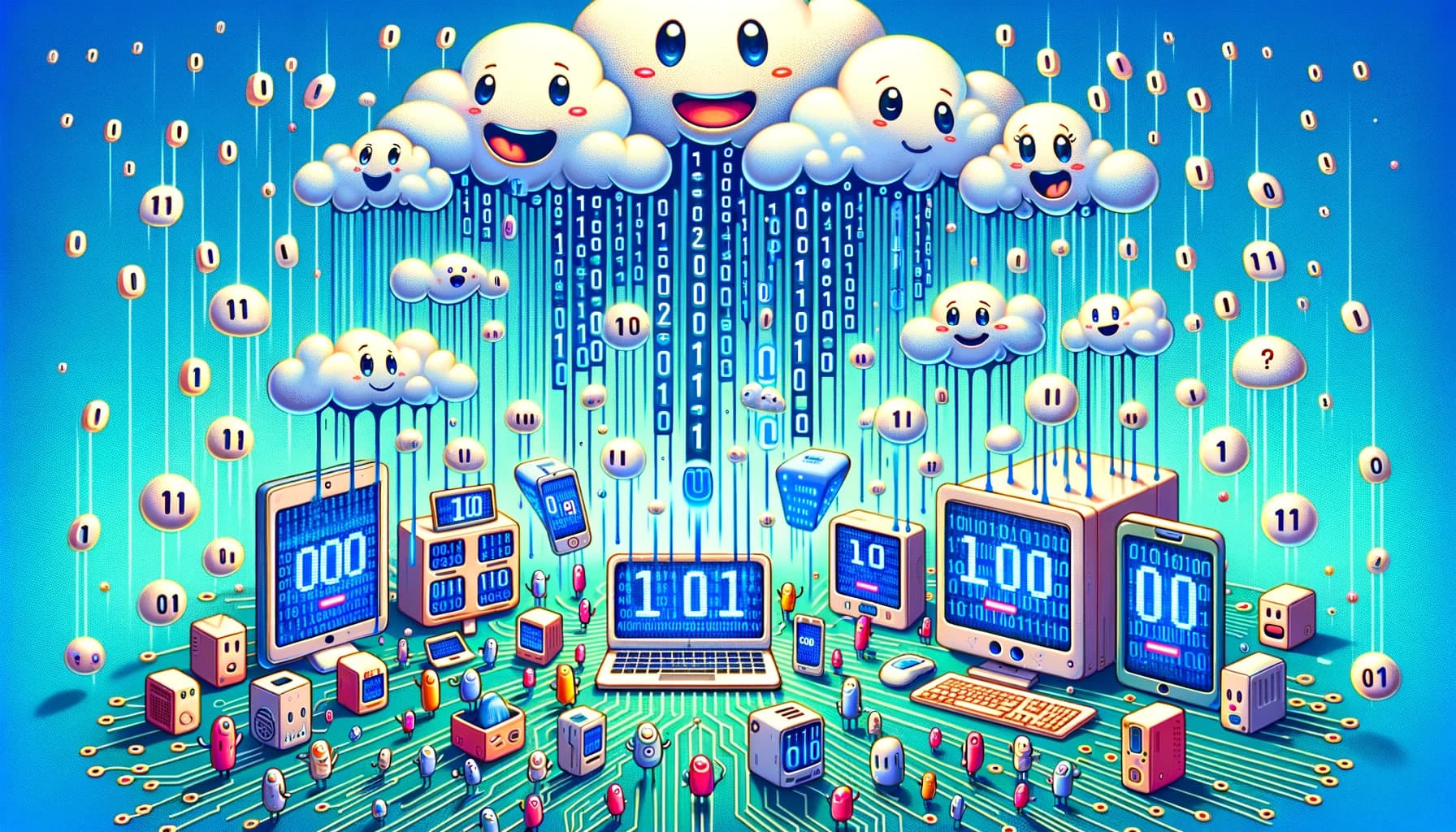
In the vast expanse of the developer's universe, where data streams flow as endless as the stars, there exists a realm where only the bravest coders dare to venture: the dimension of binary I/O operations. Lab 5: Binary I/O Files is your spacecraft in this odyssey, equipped with the sophisticated machinery of C++ to explore the mysterious depths of binary file handling, piloting through the nebulae of ShipInfo
and WeaponType
data with precision and grace.
For source code please visit the Github repository here: Binary IO Files
Overview
Lab 5 focuses on demonstrating binary input/output (I/O) operations in C++, using a practical example of managing spaceship data. The project involves reading from and writing to binary files (friendlyships.shp
and enemyships.shp
), showcasing how to handle complex data structures in a binary format.
Repository Structure
main.cpp
: The primary C++ source file containing the logic for reading from and writing to binary files, as well as the implementation of theShipInfo
andWeaponType
classes.main.h
: Header file formain.cpp
, potentially intended to declare theShipInfo
andWeaponType
classes and any necessary functions.enemyships.shp
andfriendlyships.shp
: Binary files containing data for enemy and friendly ships, respectively. These files serve as input for the project to demonstrate binary file reading capabilities.Lab 5.pdf
: Documentation providing an overview and specifications for the binary I/O operations project.
Key Functionalities
ShipInfo and WeaponType Classes
- ShipInfo: Represents information about a ship, including name, class, length, shield capacity, warp speed, and the number of weapons.
- WeaponType: Represents a weapon equipped on a ship, including the weapon's name, power level, and version.
Binary File Operations
- Reading from Binary Files: The application reads ship and weapon data from binary files, demonstrating how to deserialize complex data structures.
- Writing to Binary Files: While the provided content does not explicitly include writing operations, the principles demonstrated in reading operations can be applied inversely for writing to binary files.
User Interaction
- The application prompts users to choose between reading enemy ship data, friendly ship data, or both. It then allows users to select an action to perform on the loaded data, such as listing all ships, finding the starship with the strongest weapon, and more.
Usage
- Compile the
main.cpp
file using a C++ compiler that supports C++11 or later. - Run the compiled application. Follow the on-screen prompts to select which binary files to read and what operations to perform on the loaded data.
Example Operations
// Example function call to load ship data from a binary file
openfile("friendlyships.shp", ships, weapons);
// Example choices provided to the user for interacting with the loaded data
cout << "1. Print all ships" << endl;
cout << "2. Starship with the strongest weapon" << endl;
cout << "3. Strongest starship overall" << endl;
cout << "4. Weakest ship (ignoring unarmed)" << endl;
cout << "5. Unarmed ships" << endl;
Conclusion
As our journey through Lab 5: Binary I/O Files concludes, we find ourselves not just as observers of binary stars but as navigators and chroniclers of their mysteries. This expedition has not only demonstrated the power of C++ in manipulating the fabric of binary data but has also illuminated the pathways to efficient and performant data storage and retrieval. Armed with the knowledge of binary operations, we are now custodians of a universe where data structures and algorithms coalesce into the art of programming. So, fellow travelers, with these insights etched into the annals of your coder's log, what new frontiers will you explore in the binary cosmos?