Showroom Showdown: Crafting C++ Classes for the Ultimate Dealership Duel
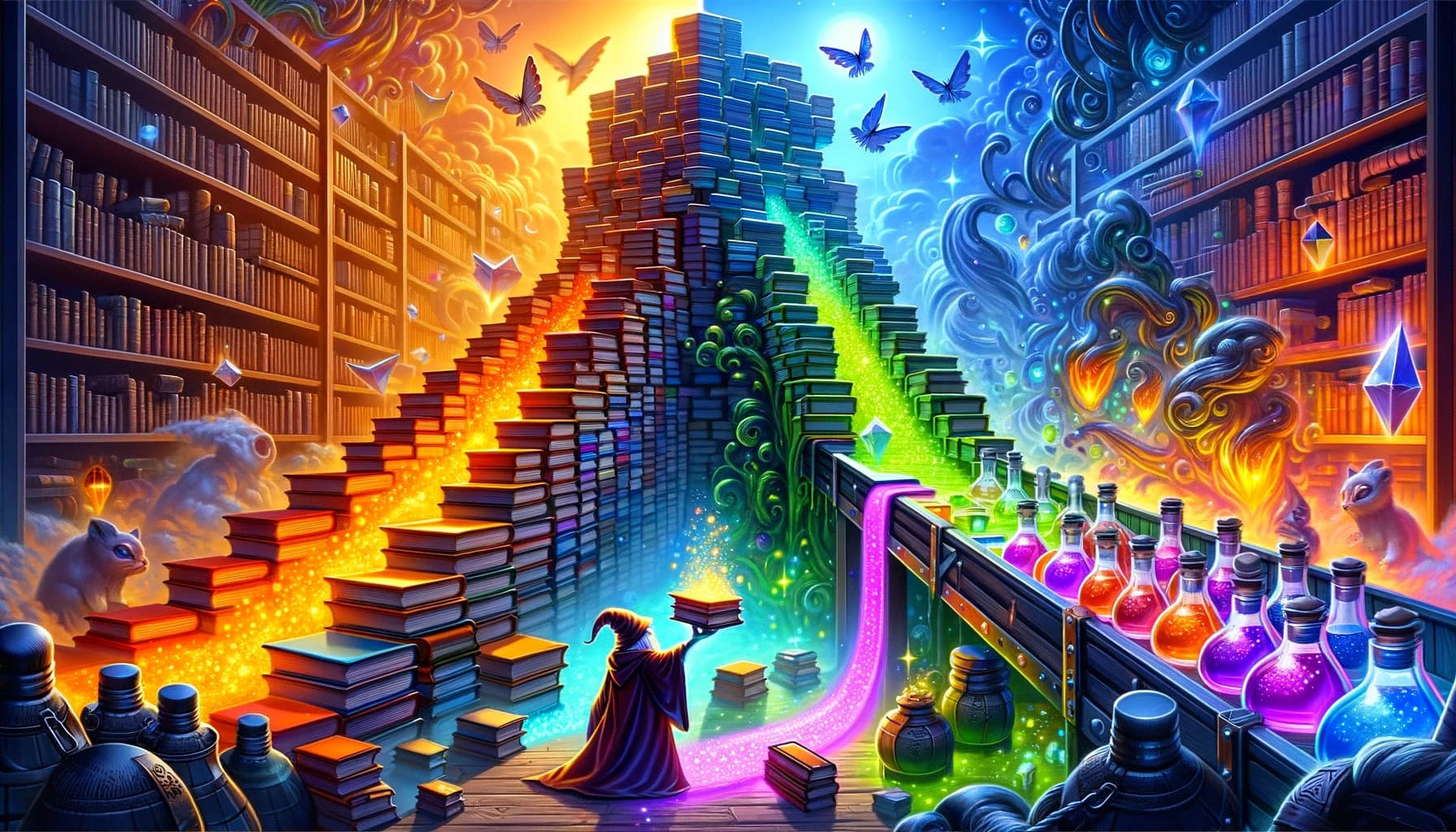
In the garage of programming, where ideas take shape like cars on an assembly line, there's a project that stands out on the showroom floor: Lab 1: Classes. This is where the rubber meets the code, blending the art of object-oriented programming with the precision of C++. Get ready to engineer a dealership management system that's as robust as a truck yet as sleek as a sports car, all through the power of classes, objects, and aggregation.
For source code please visit the Github repository here: ABS_ABQ
Overview
Lab 1: Classes is a C++ project designed to illustrate the fundamental concepts of object-oriented programming, including class definition, object instantiation, and basic operations on objects. The project simulates a simple dealership management system, manipulating vehicles and showrooms.
Repository Structure
- Lab 1.pdf: Documentation providing an overview and specifications for the lab project.
- Dealership.cpp & Dealership.h: Defines the Dealership class, managing a collection of Showroom objects.
- Main.cpp & Main.h: Contains the main driver code that demonstrates the usage of Vehicle, Showroom, and Dealership classes.
- Showroom.cpp & Showroom.h: Implements the Showroom class, managing a collection of Vehicle objects.
- Vehicle.cpp & Vehicle.h: Defines the Vehicle class, representing individual vehicles with attributes like make, model, year, price, and mileage.
Key Functionalities
Vehicle Class
The Vehicle class encapsulates data about a vehicle, including its make, model, year, price, and mileage. It provides methods to display vehicle information and access vehicle attributes.
- Constructor Overloading: Supports creating a Vehicle object with default values or specified attributes.
- Display Information: A method to neatly print the vehicle's details.
Showroom Class
The Showroom class represents a showroom that can hold multiple vehicles. It allows adding vehicles to the showroom and displays all vehicles within it.
- Capacity Management: Limits the number of vehicles in a showroom based on its capacity.
- Add Vehicle: Adds a vehicle to the showroom if it has not reached its capacity.
- Show Inventory: Lists all vehicles in the showroom, including their details.
Dealership Class
The Dealership class manages multiple showrooms. It demonstrates the aggregation relationship in OOP, where a dealership aggregates several showrooms.
- Add Showroom: Incorporates a new showroom into the dealership.
- Show Inventory: Displays the inventory of all showrooms under the dealership, including each vehicle's details.
- Average Price Calculation: Computes the average price of all vehicles across all showrooms in the dealership.
Example Usage
The main function (Main.cpp
) demonstrates the usage of these classes through a series of tests:
- Creating Vehicles: Instantiates Vehicle objects with different attributes.
- Managing a Showroom: Adds vehicles to a showroom and displays the showroom inventory.
- Dealership Operations: Creates a dealership, adds showrooms to it, and displays the entire dealership's inventory.
Conclusion
As we park Lab 1: Classes in the garage of our coding journey, we step out not just as programmers, but as maestros of object-oriented design. This venture into the world of C++, dealership management, and class construction is more than a lesson; it's a launching pad into the realms of software development, where the constructs of our imagination fuel the innovations of tomorrow. So, gear up, coders, for the roads ahead are paved with endless possibilities, waiting for you to hit the gas on your next big idea.